📃 需求描述
项目在开发时需要进行多环境部署打包,每个环境间的配置信息并不互通,若要进行人为的手动替换,不但增加了机械且额外的工作量,还容易出错,因此需要通过配置 Maven,使得能够为不同环境打包不同的配置信息。
🙋 解决方案
🗃 resources 目录
📁 目录配置
在 resource 目录下添加 env 目录,用于放置不同的环境,如开发环境、测试环境,在 env 下创建 dev、test 目录即可。
1 2 3 4
| resources └── env ├── dev └── test
|
当然,文件名可以为任意有效字符,但需要语义清楚,并与 pom 中指定路径相对应。
⚙ Maven 配置
在 pom 中添加环境属性,与 env 中创建的环境目录相应。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| <profiles> <profile> <id>dev</id> <build> <resources> <resource> <directory>src/main/resources/env/dev</directory> </resource> </resources> </build> <activation> <activeByDefault>true</activeByDefault> </activation> </profile> <profile> <id>test</id> <build> <resources> <resource> <directory>src/main/resources/env/test</directory> </resource> </resources> </build> </profile> </profiles>
|
此时,在 idea 侧边的 maven 插件中的 Profiles 已经可以看到可选环境。
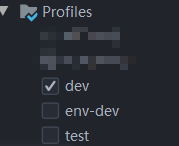
🔧 细节处理
此处会将 env 目录也一同打包,但实际上在这打包后已经是无用文件了,因此需要将其剔除。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| <build> <resources> <resource> <directory>src/main/resources</directory> <filtering>true</filtering> <excludes> <exclude>**/env/**</exclude>
<exclude>application-xxx.yml</exclude> </excludes> </resource> </resources> </build>
|
📰 webapps 目录
📁 目录配置
同样,需要现在 webapps 目录下为不同的环境配置不同的文件路径,如下 prod 、test 分别表示生产和测试环境。
1 2 3 4
| webapps └── env ├── prod └── test
|
⚙ Maven 配置
与之前的不同的是,webapps 下面的文件需要借助 maven-war-plugin 插件。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-war-plugin</artifactId> <configuration> <warSourceExcludes> env/test/**,env/prod/** </warSourceExcludes> <webResources> <resource> <directory>src/main/webapp/env/${active}</directory> <targetPath>/</targetPath> <filtering>true</filtering> </resource> </webResources> </configuration> </plugin>
|
🚩 注意
为了实现多环境配置,此处在 directory 中,使用了变量 active 来动态匹配路径。至于其值则是通过各个环境的配置文件定义的。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <profiles> <profile> <id>test</id> <properties> <active>test</active> </properties> </profile> <profile> <id>prod</id> <properties> <active>prod</active> </properties> <activation> <activeByDefault>true</activeByDefault> </activation> </profile> </profiles>
|
此处并没有为每个环境直接指定路径,而是设置了一个 key 为 active 的属性,其 value 对应各自环境的文件夹名。